Resolva Recaptcha V2
Esse tipo de tarefa resolve Google Recaptcha V2 sem proxy. A tarefa será executada usando nossos próprios servidores de proxy e/ou endereços de IP dos workers.
A tarefa é executada usando nossos próprios servidores de proxy e/ou endereço de IP dos nossos workers. No momento, o Recaptcha não possui proteção de situações onde um quebra-cabeça é resolvido em um endereço de IP e o formulário com a g-response é enviado de um outro. A API da Google não fornece e o endereço de IP da pessoa que resolveu seu Recaptcha. Se isso mudar, você sempre poderá uar nosso tipo de tarefa padrão - RecaptchaV2Task.
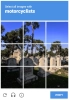
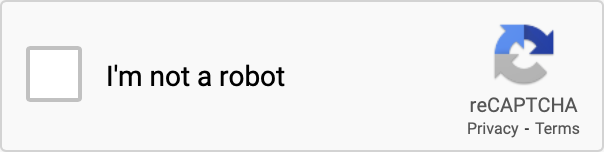
Exemplo do Recaptcha V2
Objeto da tarefa
Propriedade | Tipo | Obrigatório | Motivo |
---|---|---|---|
type | String | Sim | RecaptchaV2TaskProxyless Nome anterior do tipo de tarefa: NoCaptchaTaskProxyless. Ele sempre será suportado, não precisa atualizar o seu código. |
websiteURL | String | Sim | Endereço de uma página web de interesse. Pode estar localizado em qualquer lugar no website, até mesmo na área de membro. Nossos workers não navegam em tais lugares, mas simulam a visita. |
websiteKey | String | Sim | Chave de website do Recaptcha. Descubra como encontrá-la neste artigo. |
recaptchaDataSValue | String | Não | Valor do parâmetro 'data-s'. É usado apenas para Recaptchas em um website do Google. |
isInvisible | Boolean | Não | Especifica se o Recaptcha está invisível. Isso vai renderizar um widget apropriado para nossos workers. |
Exemplo de solicitação
Python
Javascript
Go
PHP
Java
C#
bash
#pip3 install anticaptchaofficial
from anticaptchaofficial.recaptchav2proxyless import *
solver = recaptchaV2Proxyless()
solver.set_verbose(1)
solver.set_key("YOUR_API_KEY_HERE")
solver.set_website_url("https://website.com")
solver.set_website_key("SITE_KEY")
#set optional custom parameter which Google made for their search page Recaptcha v2
#solver.set_data_s('"data-s" token from Google Search results "protection"')
# Specify softId to earn 10% commission with your app.
# Get your softId here: https://anti-captcha.com/clients/tools/devcenter
solver.set_soft_id(0)
g_response = solver.solve_and_return_solution()
if g_response != 0:
print("g-response: "+g_response)
else:
print("task finished with error "+solver.error_code)
Objeto de solução de tarefa
Propriedade | Tipo | Motivo |
---|---|---|
gRecaptchaResponse | String | String de token que é necessária para interagir com o formulário de envio no website de interesse. |
cookies | Array | Array opcional de cookies que foram usados para a resolução do Recaptcha. Usando apenas para domínios e subdomínios google.com. |
Exemplo de resposta
{
"errorId":0,
"status":"ready",
"solution":
{
"gRecaptchaResponse":"3AHJ_VuvYIBNBW5yyv0zRYJ75VkOKvhKj9_xGBJKnQimF72rfoq3Iy-DyGHMwLAo6a3"
},
"cost":"0.001500",
"ip":"46.98.54.221",
"createTime":1472205564,
"endTime":1472205570,
"solveCount":"0"
}