Solve GeeTest captcha
This type of task solves GeeTest captchas in our workers' browsers. Your app submits the website address, gt key, challenge key and after task completion receives a solution consisting of 3 tokens. For version GeeTest version 4 output consists of 5 values and challenge key is not required.
Everything is similar to GeeTestTask, except we don't require proxy and we solve it from our own IP addresses.
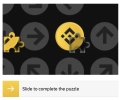
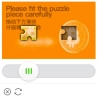
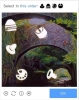
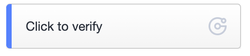
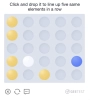
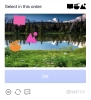
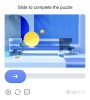
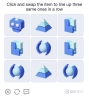
Examples
Task object
Related tutorial: Learn how to use breakpoints in Chrome to find API parameters for FunCaptcha and Geetest
Property | Type | Required | Purpose |
---|---|---|---|
type | String | Yes | GeeTestTaskProxyless |
websiteURL | String | Yes | Address of a target web page. Can be located anywhere on the web site, even in a member area. Our workers don't navigate there but simulate the visit instead. |
gt | String | Yes | The domain public key, rarely updated. |
challenge | String | No | Changing token key. Make sure you grab a fresh one for each captcha; otherwise, you'll be charged for an error task. Required for version 3. Not required for version 4 |
geetestApiServerSubdomain | String | No | Optional API subdomain. May be required for some implementations. ![]() |
version | Integer | No | Version number. Default version is 3. Supported versions: 3 and 4. |
initParameters | Object | No | Additional initialization parameters for version 4 |
Request example (V3)
Python
Javascript
PHP
Java
C#
bash
#pip3 install anticaptchaofficial
from anticaptchaofficial.geetestproxyless import *
solver = geetestProxyless()
solver.set_verbose(1)
solver.set_key("YOUR_API_KEY_HERE")
solver.set_website_url("https://address.com")
solver.set_gt_key("CONSTANT_GT_KEY")
solver.set_challenge_key("VARIABLE_CHALLENGE_KEY")
# optional API subdomain, make sure you understand what to set here
# solver.set_js_api_domain("custom-domain.geetest.com")
# getLib payload, see documentation for explanation of this
# solver.set_geetest_lib("{\"customlibs\":\"url-to-lib.js\"}")
# Specify softId to earn 10% commission with your app.
# Get your softId here: https://anti-captcha.com/clients/tools/devcenter
solver.set_soft_id(0)
token = solver.solve_and_return_solution()
if token != 0:
print "result tokens: "
print token
else:
print "task finished with error "+solver.error_code
GeeTest V4
Python
Javascript
PHP
Java
C#
bash
#pip3 install anticaptchaofficial
from anticaptchaofficial.geetestproxyless import *
solver = geetestProxyless()
solver.set_verbose(1)
solver.set_key("YOUR_API_KEY_HERE")
solver.set_website_url("https://address.com")
solver.set_gt_key("captchaId value")
solver.set_version(4)
# optional API subdomain, make sure you understand what to set here
# solver.set_js_api_domain("custom-domain.geetest.com")
# optional initialization parameters
# solver.set_init_parameters({"riskType": "slide"})
# Specify softId to earn 10% commission with your app.
# Get your softId here: https://anti-captcha.com/clients/tools/devcenter
solver.set_soft_id(0)
token = solver.solve_and_return_solution()
if token != 0:
print "result tokens: "
print token
else:
print "task finished with error "+solver.error_code
Task solution object (V3)
Property | Type | Purpose |
---|---|---|
challenge | String | Hash string required for interacting with the submit form on the target website. |
validate | String | Hash string which is also required. |
seccode | String | Another required hash string; we have no idea why there are 3 of them. |
Task solution object (V4)
Property | Type |
---|---|
captcha_id | String |
lot_number | String |
pass_token | String |
gen_time | Integer |
captcha_output | String |
Response example (v3)
{
"errorId":0,
"status":"ready",
"solution":
{
"challenge":"3c1c5153aa48011e92883aed820069f3hj",
"validate":"47ad5a0a6eb98a95b2bcd9e9eecc8272",
"seccode":"83fa4f2d23005fc91c3a015a1613f803|jordan"
},
"cost":"0.001500",
"ip":"46.98.54.221",
"createTime":1472205564,
"endTime":1472205570,
"solveCount":"0"
}
Response example (v4)
{
"errorId":0,
"status":"ready",
"solution":
{
"captcha_id": "fcd636b4514bf7ac4143922550b3008b",
"lot_number": "354ab6dd4e594fdc903074c4d8d37b24",
"pass_token": "b645946a654e60218c7922b74b3b5ee8e8717e8fd3cd5182a5c98d660bbd1ed5",
"gen_time": "1649921519",
"captcha_output": "cFPIALDXSop8Ri2mPABbRWzNBs86N8D4vNUTuVa7wN7E...[cut]...ciM50ePCCzLBZ1bmaV9Yt7IkkFI9Emx4eaP8rRoA=="
},
"cost":"0.001500",
"ip":"46.98.54.221",
"createTime":1472205564,
"endTime":1472205570,
"solveCount":"0"
}