Bypass Google Recaptcha V2 Enterprise without proxy
This type of task is for solving Google Recaptcha Enterprise V2 from the worker's IP address.
It is mostly similar to RecaptchaV2TaskProxyless, except tasks are solved using the Enterprise API and assigned to workers with the best reCaptcha V3 score.
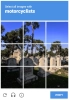
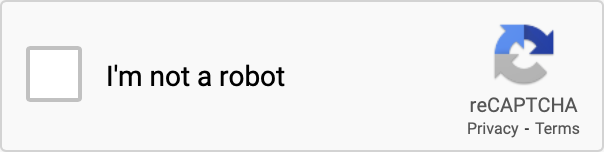
Recaptcha V2 Enterprise example. Visually, it is identical to the non-enterprise version.
Task object
Property | Type | Required | Purpose |
---|---|---|---|
type | String | Yes | RecaptchaV2EnterpriseTaskProxyless |
websiteURL | String | Yes | Address of a target web page. Can be located anywhere on the web site, even in a member area. Our workers don't navigate there but simulate the visit instead. |
websiteKey | String | Yes | Recaptcha website key. Learn how to find it in this article. |
enterprisePayload | Object | No | Additional parameters which should be passed to "grecaptcha.enterprise.render" method along with sitekey. Example of what you should search for: grecaptcha.enterprise.render("some-div-id", {
sitekey: "6Lc_aCMTAAAAABx7u2N0D1XnVbI_v6ZdbM6rYf16",
theme: "dark",
s: "2JvUXHNTnZl1Jb6WEvbDyBMzrMTR7oQ78QRhBcG07rk9bpaAaE0LRq1ZeP5NYa0N...ugQA"
}); |
apiDomain | String | No | Use this parameter to send the domain name from which the reCaptcha script should be served. Can have only one of two values: "www.google.com" or "www.recaptcha.net". Do not use this parameter unless you understand what you are doing. |
Request example
Python
Javascript
PHP
Java
C#
bash
#pip3 install anticaptchaofficial
from anticaptchaofficial.recaptchav2enterpriseproxyless import *
solver = recaptchaV2EnterpriseProxyless()
solver.set_verbose(1)
solver.set_key("YOUR_API_KEY_HERE")
solver.set_website_url("https://website.com")
solver.set_website_key("YOUR_API_KEY_HERE")
# solver.set_enterprise_payload({"s": "sometoken"})
# Specify softId to earn 10% commission with your app.
# Get your softId here: https://anti-captcha.com/clients/tools/devcenter
solver.set_soft_id(0)
g_response = solver.solve_and_return_solution()
if g_response != 0:
print("g-response: "+g_response)
else:
print("task finished with error "+solver.error_code)
Task solution object
Property | Type | Purpose |
---|---|---|
gRecaptchaResponse | String | Token string required for interacting with the submit form on the target website. |
Response example
{
"errorId":0,
"status":"ready",
"solution":
{
"gRecaptchaResponse":"3AHJ_VuvYIBNBW5yyv0zRYJ75VkOKvhKj9_xGBJKnQimF72rfoq3Iy-DyGHMwLAo6a3"
},
"cost":"0.001500",
"ip":"46.98.54.221",
"createTime":1472205564,
"endTime":1472205570,
"solveCount":"0"
}