Solve Turnstile captcha via a proxy
Turnstile captcha is another attempt to replace reCaptcha. We support all its subtypes automatically: manual, non-interactive, and invisible. No need to specify the subtype. Also, providing your own custom User-Agent is not necessary and won't work at all.
This type of task requires a proxy. Please use it only if proxy-off tasks (TurnstileTaskProxyless) are failing, as it slows down our workers. Solving captchas with proxies also requires super high quality of your proxies which you should install yourself on your own VPS servers and never use purchased proxy services.
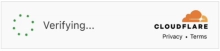
Captcha example
Task object
Property | Type | Required | Purpose |
---|---|---|---|
type | String | Yes | TurnstileTask |
websiteURL | String | Yes | Address of a target web page. Can be located anywhere on the web site, even in a member area. Our workers don't navigate there but simulate the visit instead. |
websiteKey | String | Yes | Turnstile sitekey |
action | String | No | Optional "action" parameter. |
cData | String | No | Optional "cData" token |
chlPageData | String | No | Optional "chlPageData" token |
proxyType | String | Yes | Type of proxy http - usual http/https proxy socks4 - socks4 proxy socks5 - socks5 proxy |
proxyAddress | String | Yes | Proxy IP address ipv4/ipv6. No host names or IP addresses from local networks. |
proxyPort | Integer | Yes | Proxy port |
proxyLogin | String | Yes | Login for proxy which requires authorization (basic) |
proxyPassword | String | Yes | Proxy password |
Request example
Python
Javascript
Go
PHP
Java
C#
bash
#pip3 install anticaptchaofficial
from anticaptchaofficial.turnstileproxyon import *
solver = turnstileProxyon()
solver.set_verbose(1)
solver.set_key("YOUR_API_KEY_HERE")
solver.set_website_url("https://website.com/")
solver.set_website_key("sitekey_here")
solver.set_proxy_address("PROXY_ADDRESS")
solver.set_proxy_port(1234)
solver.set_proxy_login("proxylogin")
solver.set_proxy_password("proxypassword")
# Optionally specify page action
solver.set_action("login")
# Optionally specify cData and chlPageData tokens for Cloudflare pages
#solver.set_cdata("cdata_token")
#solver.set_chlpagedata("chlpagedata_token")
# Specify softId to earn 10% commission with your app.
# Get your softId here: https://anti-captcha.com/clients/tools/devcenter
solver.set_soft_id(0)
token = solver.solve_and_return_solution()
if token != 0:
print("token: "+token)
else:
print("task finished with error "+solver.error_code)
Task solution object
Property | Type | Purpose |
---|---|---|
token | String | Token string required for interacting with the submit form on the target website. |
userAgent | String | User-Agent of the worker's browser. Use it when you submit the response token. |
Response example
{
"errorId":0,
"status":"ready",
"solution":
{
"token":"0.vtJqmZnvobaUzK2i2PyKaSqHELYtBZfRoPwMvLMdA81WL_9G0vCO3y2VQVIeVplG0mxYF7uX.......",
"userAgent":"Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:102.0) Gecko/20100101 Firefox/102.0"
},
"cost":"0.001500",
"ip":"46.98.54.221",
"createTime":1472205564,
"endTime":1472205570,
"solveCount":"0"
}